Elixir and the Phoenix framework have been gaining more and more popularity over the last couple of years. In this post I will be giving an introduction to the language and the framework. I’ll also be discussing its benefits, why you would choose to use it and which companies are currently using it in production. Coming from a Ruby/Rails background, I will also be drawing comparisons between the two and will talk about the similarities.
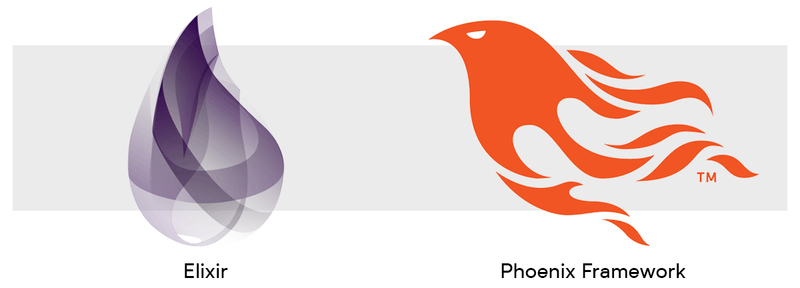
What is it?
Elixir is a functional programming language created in 2011 by José Valim, who was part of the core Ruby on Rails team. He created Elixir was as a result of an issue he discovered while improving the performance of Rails. His main struggle was finding the proper tools to solve concurrency problems. Whilst looking for a solution, he turned his eyes to other technologies and found the Erlang virtual machine. His main vision was to create a language with more constructs and excellent tooling, with similarities to the syntax of Ruby.
Companies currently using Elixir in production:
- Discord is a free voice and text chat for gamers. They used Elixir to scale up to 5,000,000 concurrency users and millions of events per second.
- Findmypast makes original historical documents available to search online, so that people can explore a collection of over two billion records find their ancestors and trace their family tree from the comfort of their own home, worldwide.
- Pinterest is using Elixir for its notification system to deliver 14,000 notifications per second. The notification system runs across 15 servers, whereas the old system, written in Java, ran on 30. The new code is about one-tenth of the size of the old code.
- Whitehat is a tech startup using Elixir along with Phoenix for its backend platform. It helps people find the best career-focused apprenticeships available with big companies such as Facebook, Google, Moo, Expedia and Warner Brothers.
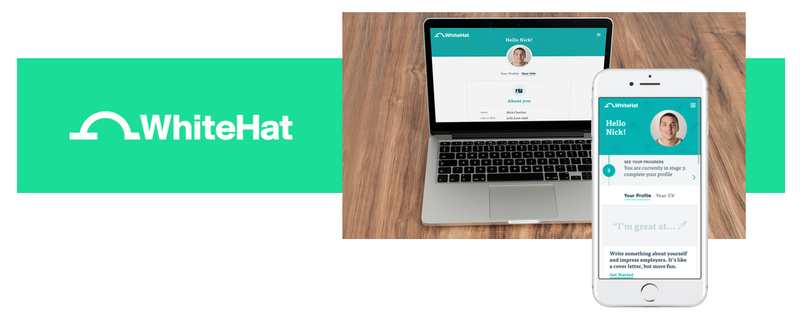
Where does it come from?
Elixir runs on top of a programming language known as Erlang VM (BEAM). It shares the same abstractions for building distributed fault-tolerant applications that are concurrent, and is used to build massively scalable, soft real-time systems that require high availability. More than 30 years old and created by Ericsson, it was originally a proprietary language that they used internally before it was open sourced in 1988. It was initially created for telecoms and computer telephony but is now used in banking, ecommerce and instant messaging.
Many large companies are currently using Erlang in production:
- Amazon uses Erlang to implement SimpleDB, providing database services as a part of the Amazon Elastic Compute Cloud (EC2).
- Yahoo! uses it in its social bookmarking service, Delicious, which has more than 5 million users and 150 million bookmarked URLs.
- Facebook uses Erlang to power the backend of its chat service, handling more than 100 million active users.
- WhatsApp uses Erlang to run messaging servers, achieving up to 2 million connected users per server.
- T-Mobile uses Erlang in its SMS and authentication systems.
- Motorola is using Erlang in call processing products in the public-safety industry.
- Ericsson uses Erlang in its support nodes, used in GPRS and 3G mobile networks worldwide.
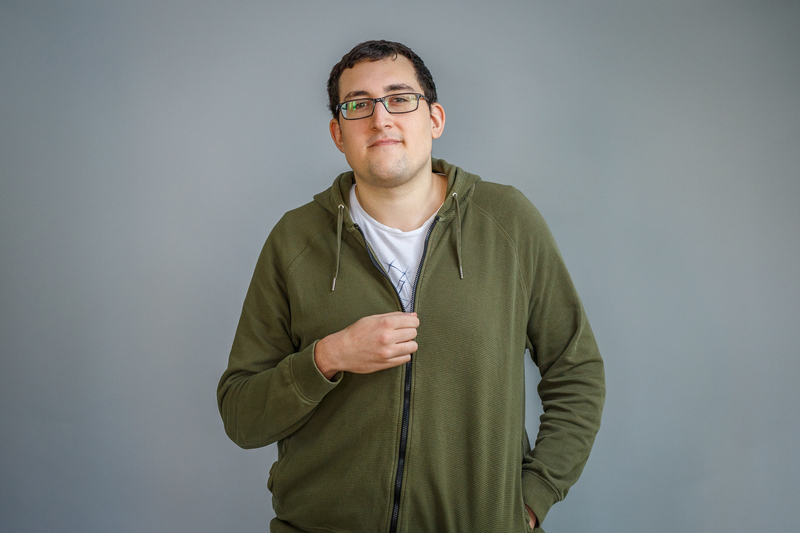
Why use it over Ruby/Rails?
Both languages have their own advantages. Ruby on Rails excels at prototyping applications because of how fast you can get a site up and ready in production. The ecosystem is large there are lots of libraries that currently solve a lot of problems and save a lot of time. Elixir and Phoenix are still quite early in their development, so are lacking some libraries, which makes the process of prototyping applications a little slower.
Elixir and Phoenix are much more suited for applications that you anticipate will have a large target user base. Because it is concurrent, it scales much more easily than Ruby on Rails. It is a compiled language, so it’s much more likely that you’ll spot bugs earlier, which can help with overall development time.
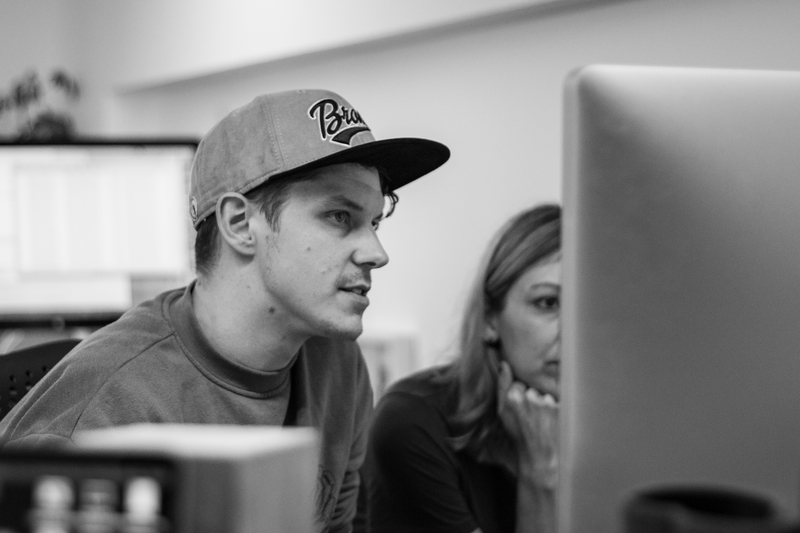
How do they compare?
Elixir and Ruby have similar build tools; ‘mix’ on the Elixir side VS ‘rake’ on the Ruby side. Likewise, ‘Hex’ package manager operates similar to ‘Gems’ with Ruby. The Phoenix Framework is heavily influenced by Ruby on Rails and also uses the MVC (Model, View, Controller) architectural pattern. The bonus on the Phoenix side is that it includes a presentation layer called ‘views’ straight out of the box, so not a lot of extra setup is needed.
My favourite parts of using Elixir and Phoenix so far have been the speed of page loads, the syntax (which is so close to Ruby) and the compilation of code (which is a form of validation) to ensure the code can run. For example:
Pattern matching
defmodule Talker do
def say_hello("Bob"), do: "Hello, Bob!"
def say_hello("Jane"), do: "Hi there, Jane!"
def say_hello(name) do
"Hi, #{name}."
end
end
In Elixir, it is possible to define the same method multiple times expecting different arguments. This allows us to pattern-match. With the example above we can do this:
Talker.say_hello("Bob") => "Hello, Bob!"
Talker.say_hello("Jane") => "Hi there, Jane!"
Talker.say_hello("Ahmet") => "Hi, Ahmet."
This is especially useful, as you can catch nil values before they hit your function.
defmodule Talker do
def say_hello(nil), do: "Please give me a name"
def say_hello(name) do
"Hi, #{name}."
end
end
In Ruby, you would return something from the same function by checking if the value is nil
class Talker
def say_hello(name)
return "Please give me a name" if name.nil?
"Hi, #{name}."
end
end
Type checking
Elixir has support for type checking, which I’ve found very useful. It again allows for a form of validation to ensure you are passing the correct values to functions.
defmodule Bob do
@spec say(String.t()) :: String.t()
def say(message) do
"Bob says: #{message}"
end
end
The above ensures the say function only ever passes a string as the first argument and returns a string. The format is:
@spec {method name}({type of parameter}) :: {type of return value}
Pipe operator
The pipe operator |>
passes the result of an expression as the first parameter of another expression. I’ve found this to be a really useful benefit over Ruby as it allows you to chain functions together and clearly see the flow.
"Elixir rocks" |> String.upcase() |> String.split()
=> ["ELIXIR", "ROCKS"]
In Ruby, this would be done by calling .upcase
then .split
on the string. This is just a basic example but being able to chain functions together, which could do anything, means you are therefore able to understand the flow of the code without having to dig around.
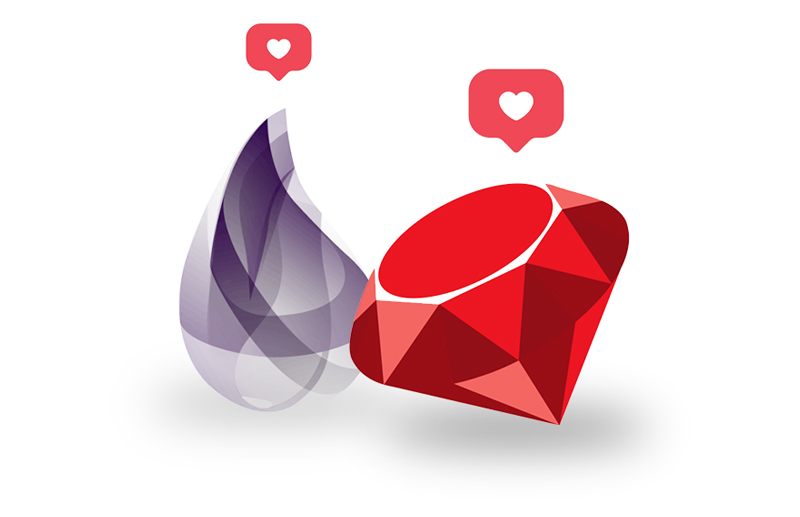
Conclusion
Both languages are great at what they do best. There is no ‘better’ option. If you are a Ruby developer and have never looked into Elixir, I would suggest you give it a go! It’s always good to learn new things and there is not a huge jump from Ruby. Have a run through ElixirSchool, which provides the best overview for the language. It covers the basics all the way up to the more advanced sections of Elixir. I hope this blog article helped spark your interest in the language, and thanks for reading.